Make your own Tower Defense Game with PyGame
In this course you’ll learn how to write a 2d Tower Defense Game from scratch, using PyGame. Writing a mess of spaghetti code is easy; but writing code that is maintainable and easy to extend is not. A tower defense game is a perfect place to learn how to write a substantial game that will test your skills as a Python programmer. It is also a perfect template for many other 2d games.
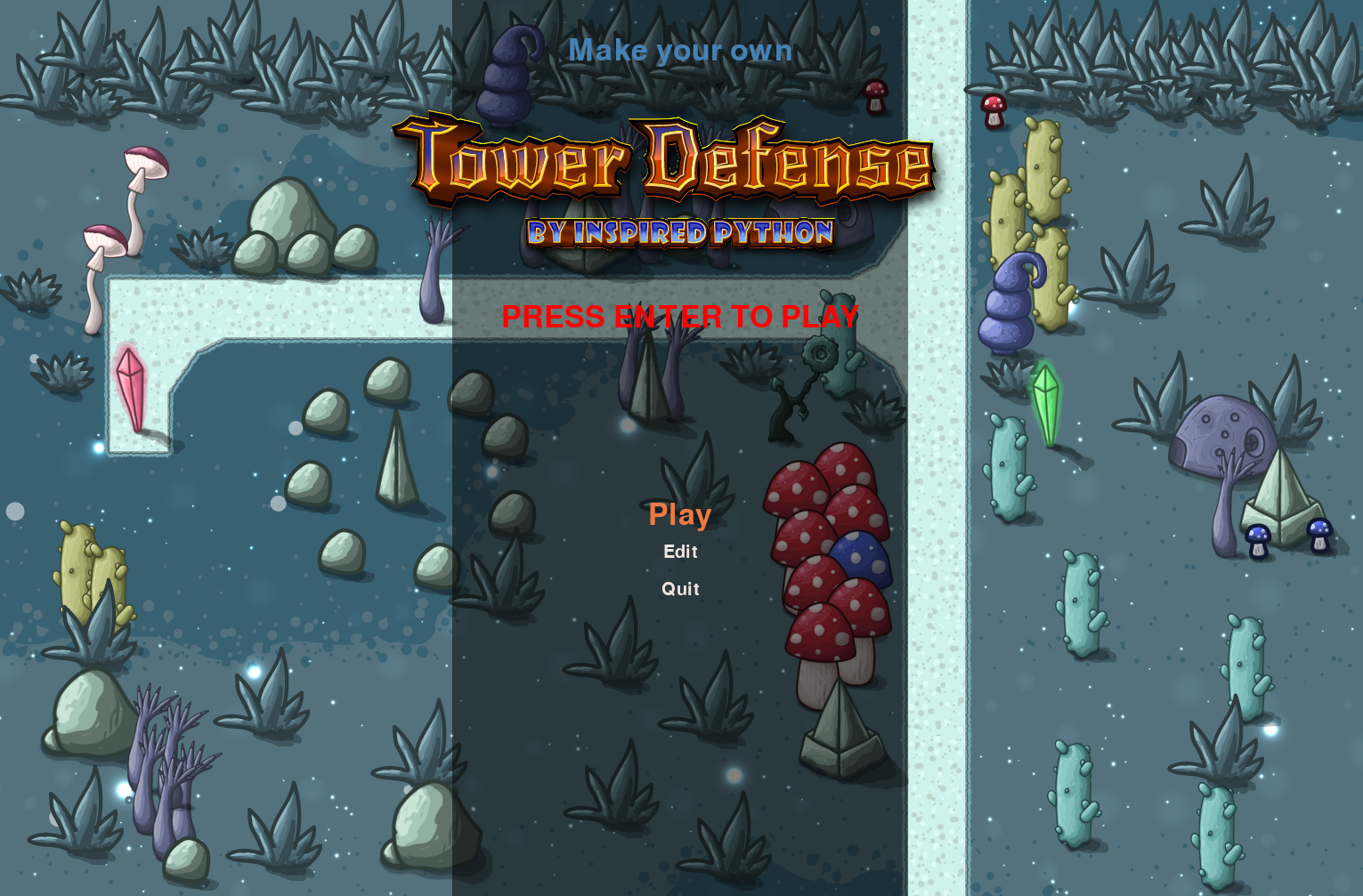
Part of the challenge of writing a game is the many disparate disciplines that rear their heads once you move beyond the truly basic. During the course you’ll learn the following skills:
- What is a Game Loop?
-
How do games actually update and display things on the screen in a manner that is maintainable and easy to reason about for the budding game developer?
The Game Loop is a cornerstone of all games, big and small. You’ll learn how to create one, and how it’s used to handle keyboard and mouse input, graphics rendering, updating the physics of entities on the screen, and more.
- State Machines and Transitions
-
Few games have just one screen, and thus one state. Most games have a main menu, a score board, the actual game, and possibly more states that a player interacts with during game play. Understanding how to transition your game’s state between these different concepts is critical to writing a game free of spaghetti code.
You’ll learn about finite-state machines, an important concept in Computer Science, and how it can easily transform a complex set of confusing requirements into neat and tidy code.
- Lazy evaluation, Generators and Iterables
-
Keeping track of the position of things – and calculating the next position of something, such as a flying bullet – is easily solved with a liberal use of Python’s
itertools
library and generators.Master a part of Python that gets short thrift from most developers, as generators use lazy evaluation to only calculate the next thing you need.
- Drawing and manipulating graphics
-
Learn what a sprite is. Sprites are the one of the fun parts of game development: the graphics. They’re most things you’ll see and interact with on your screen. You’ll learn how to make ‘em move smoothly across the screen; scale them up or down, so you can vary their size; and rotate them, so you can make a cannon ball spin in the air as it moves.
- Level Editing
-
You’ll write a complete level editor capable of placing and editing all the entities that make up a tower defense game using a simple UI you will build yourself.
The level editor forms a core part of the game, and includes details on how to write a save and load feature, so you can share your levels with friends.
- Path finding and Recursion
-
Learn about recursion, a powerful programming concept, to find valid paths through a map for the enemies to traverse. You’ll learn about basic graph theory and how Depth-First Search is used to traverse a map and find a route from start to end.
- Vector Mathematics
-
Come to grips with the mathematics required to ensure that: a bullet travels in a straight line towards a target; that your enemies walk smoothly across the map; and how to do simple animations using nothing more than basic arithmetic.
You’ll learn about simple vector arithmetic, interpolation, and basic affine transformations (like scaling and rotating).
- Object-Oriented Programming (OOP)
-
Improve your understanding of classes and objects and how to best leverage inheritance, the factory pattern, and Python’s dataclasses to succinctly describe your game world using primitives that have applications in nearly everything you’ll ever write in Python.
- Animation
-
Learn how to chain together frames of images into simple animations so the enemies walk across the screen and collapse when they’re struck by exploding projectiles.
- Collision Detection
-
Chucking boulders and firing bullets is all well and good, but we’ll want them to do something when they strike a target. Collision detection can be hard to get right, as it has to be good enough for the game to feel like it’s fair, but also fast enough so it does not slow down your game.
Are you ready? Let’s code!
Introduction & Course Plan
So, before we start writing code in earnest, let’s take a look at the course plan, and thus what you’ll learn.
Course Plan
-
Introduction and Course Plan
-
Course Plan
-
Format of the course
-
Configuring your Python environment
-
Creating a simple Python package to host our game
-
Installing and running the demo game
-
Media assets for the game
-
-
The Game Loop Controller and Initializing PyGame
-
State Machines: How do they work, and what do they do?
-
Building a template for our game (that you can re-use for other projects, too)
-
Sprites, Sprite handling and how to interact with them.
-
Writing a 2d Tile Engine and Map Editor
-
Sprite Animation, Kinematics, and Vector Mathematics
-
Path Finding and the basics of recursion
-
Collision Detection and how to use masks
-
Menus, Sound, Text, and Wrapping up.
This is the course plan and how we’ll proceed from here on. I’ve made a special effort to ensure that I introduce things at the rate I’d expect you to add them to the game, and not necessarily in the order you’d write them in yourself, if you were to sit down and write your first-ever game. And that, I think, is a salient point I want to repeat: drawing stuff to the screen is a little while away, as I think it’s critical that we lay a good foundation before proceeding.
Required Knowledge
I’ll briefly cover what I think you should know before you begin. It’s not an iron clad rule that you know this, but it’ll make the course more approachable.
- A basic understanding of Python
-
Things like Lists, loops, dictionaries, classes, functions, and so on. You don’t have to be an object-oriented programming maven, so I don’t expect you to walk around and casually namedrop “flyweight patterns” and “abstract factories.” Ideally, all you’ll need is enough knowledge to understand what a class is, and what an object is.
We’ll write our own Python package, which is mostly a case of being mindful of the details. But knowing how to install packages with
pip
and any other supplementary requirements your platform may have (this is mostly relevant to Linux users.) OK, look, this is really quite a boring subject. But it’s there anyway. Not all game development’s fun, and there’s nothing worse than building a game and then having endless trouble getting it to run on someone else’s machine. That is most definitely not fun. - Basic mathematics
-
Yep. Mathematics. There’s no escaping it. Mostly it’s arithmetic, but we will veer into the topic of vector mathematics — but the only the basics. If you’re comfortable with simple cartesian coordinate systems and adding and subtracting numbers, you’ll do fine.
And that’s pretty much it. You don’t need any prior understanding of either PyGame or game development; but you do need a desire to want to learn it!
Graphical Assets
This game comes with high-quality graphics that are ready to use. You can find them in the demo, and more on both in a moment.
Format of the Course
This course is not a like-for-like repeat of the source code you’ll find in the game demo, but I do explain all aspects of it, so at the end of this course, you’ll have a complete understanding of everything you need to write a tower defense game (and many other 2d games!) or sit down and modify everything in the supplied demo. The supplied demo is fully working; it has assets, sound effects, a working level editor and game play. It also demonstrates everything you’ll read in this course, so you’re encouraged to refer back to it if you are stuck, or prefer looking over a complete solution.
You can approach this course in several ways, each with its own learning path, depending on what you prefer:
-
You can take the demo and start tinkering and use it as a baseline or serve as inspiration for your own game projects.
This is great if you want to learn by modifying. I really do recommend starting from scratch at some point, as you’ll learn how these things work at a deeper level than you would if you just jumped right in.
Having said that… the game engine in the demo is more than capable of – with some crafty code editing by you – of serving as a platform for other types of games. You could make the monster a main character and make it walk around with your arrow keys instead of making a tower defense game. Perfect for a simple shooter game or the trappings of an RPG. (How do to that, though, is left as an exercise to you, the reader, but if you approach the course from scratch, you’ll probably tease out how to do it along the way.)
-
You can use this course as a reference and refer back to it only when you need advice with specific parts, and reinforce your learnings with the supplied demo.
-
You can follow the course and implement each thing, one step at a time, experimenting along the way.
Or perhaps a combination of the above — the choice is yours.